Introduction To Programming In JavaScript
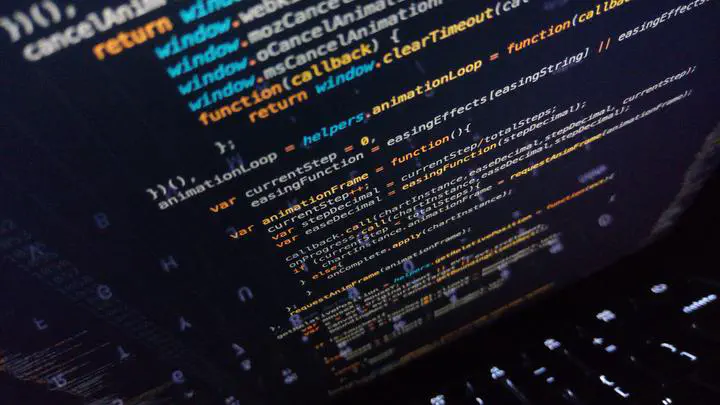
I began writing this post about 3 years ago, when I started my first blog. I wanted to consolidate my own knowledge, and provide an initial start for others who want to build their own simple websites, games and web applications. I plan to do a deep dive into the essential “building blocks” that make up all programs, so that you can go ahead and put them together to create whatever you want, just like the pros! I will cover key operators, control flow, functions, objects and arrays.
JavaScript (or ECMAScript) is a dynamically typed scripting language used to build the interactive bits of websites. You probably execute JavaScript code in your web browser every day without even realising! It was the first programming language I ever learned, and I think it’s a nice place to get started with code.
There are many languages that claim to be “simple” and “powerful”, and that advertise themselves as “beginner friendly”, so why should you start with JavaScript? Well, It was the first language I got to grips with, and I found that even though it has some quirks which can be annoying on occasion, it has powerful features, is widely used, and lets you get started quickly!
Getting Started
JavaScript makes it very easy to get going. All you need for now is a web browser, so if you’re reading this on a computer, you’re all set! You can usually access your browser’s JavaScript console by right-clicking on any page, and clicking “Inspect” (or something like that).
While this works on any page, it might be handy to do it on a blank one, which you can usually get by navigating to about:blank
. I recommend Firefox, Safari or a Chromium-based (Google Chrome, Brave, Opera, etc.) web browser for best results. Having said that, any modern browser should work fine.
Hello World!
Originally attributed to Brian Kernighan (one of the creators of the “C” language) when he worked at Bell Labs, pretty much every programmer starts off with this infamous program 1. In almost every language, this is how you output the state of your program, letting you see what’s going on “under the bonnet” so you can fix bugs.
Write the following in your JavaScript console:
console.log("hello, world");
Great! You’ve just written your first line of code. It doesn’t do much, but everyone has to start somewhere! Let’s unpack it.
You are calling the .log()
method on the console
object. You pass a string of text as a parameter, "hello, world"
. Note the line ends with ;
, like every statement in JavaScript.
You should see two things in your console. The first is the return value, undefined
, which we can ignore for now. The second is hello, world
, which is the text output by the method. How it actually does this doesn’t matter, but it essentially runs code that someone else wrote.
Comments
Comments are really handy. We use them to leave notes to describe what code is meant to do, explaining our intentions to other programmers and our future self! Some code is pretty self explanatory (2 + 3
), but sometimes you want some explanation. Comments are simply ignored by the JavaScript interpreter.
Try these out:
// Single line comment
// Another comment
// console.log("Not going to run...")
/*
Multi
line
comment
*/
Note that if you just hit return, your code typed in the console prompt will be executed immediately. If you want a new line, just hold shift as you press it. If you’ve done everything right… nothing will happen (but it may return undefined
). Well done ;)
Operations
Arithmetic
You may recognise some of these from maths! The best way to see how these work is to try them out:
Operation | Result |
---|---|
x + y | Add both numbers |
x - y | Subtract y from x |
x * y | Multiply x and y |
x / y | Divide x by y |
x % y | The remainder of dividing x by y |
Note that order of operations (BIDMAS, BODMAS, etc.) applies in programming too if you want to chain operations.
Multiplication and division may look a little weird, but the more strange to you is likely modulo (%
). Try doing 7 % 3
. The number 7
has two 3
s in it (2 * 3 = 6), with a remainder of 1
. You can do this division with 7 / 3
(2.3333333333333335), and round down the result with Math.round(7/3)
(another method). As you get more experienced, you’ll find more practical uses of this operator, but don’t worry now if it’s confusing.
Comparison
Next up are the comparison operators. Aptly named, they let you compare two values! They return true
if a match is found, or false
if not:
Operation | Result |
---|---|
x < y | Returns true if x is less than y |
x > y | Returns true if x is greater than y |
x <= y | Returns true if x is less than or equal to y |
x >= y | Returns true if x is greater than or equal to y |
x == y | Check if the values are equal, with type coercion |
x === y | Check if both the value and type are equal |
x != y | Check if the values are not equal, with type coercion |
x !== y | Check if both the value and type are not equal |
One difference you will notice is that we don’t use the normal mathematical symbols (≠, ≤ or ≥). This is probably a great relief, I highly doubt you have any of these characters on your keyboard! We also use the weird ==
sign. What does this mean? Super equals? As you’ll see, =
is the assignment operator, so we use ==
for equals.
Type Coercion?
Not unique to JavaScript, but found in other languages like PHP, a ===
is used to compare both the value and type of two items. You should almost always use this, unless you have a good reason not too. Type coercion takes two values of different types and tries to convert them to a common type to allow comparison. This can pose a security risk, so exercise caution!
In short, "3" == 3
is true, but "3" === 3
is false. The second compares both the type and value ("3"
is a string, whereas 3
is a number).
Boolean
Last but not least are the boolean operators. They allow combination of boolean expressions that are either true or false. Named after English Mathematician, George Boole 2, who came up with much of the logical mathematics that we use in computers today, long before they were even invented! That’s one smart cookie!
These operations boil down to AND (&&
), OR (||
) and NOT (!
), which allow you to chain together true
or false
logical statements, such as 2 < 3
(true) or 234 === 92
(false).
Operation | Result |
---|---|
true | Always true |
false | Always false |
A && B | True if both A and B are true |
`A | |
!A | The opposite of A |
Variables
Right, now we think about storing stuff. We’ve been manipulating values and seeing the result of this, but it would be handy to store the result for later. For this, we use variables.
We learnt earlier that JavaScript is a dynamically typed language, but what is a type, and what is this “dynamic” business about? Computers need to know the type of thing stored - is it a number, is it string of text, and so on. There are three basic types in JavaScript: string
, number
and boolean
. You can figure out the type of a value or variable using the typeof()
function.
Let’s create some variables:
var myVar = "hello";
let myLet = 3;
const myConst = true;
let aBool = false;
let aNumber = 37;
let aString = "Hey", anotherString = 'hello';
typeof(aNumber) // "number"
Note that the keywords var
and let
allow you to reassign a variable later, whereas const
creates an immutable constant, which cannot be reassigned without a TypeError
.
Scope: let, const, var
let
and const
are new(ish) in JavaScript, whereas var
is the traditional way to declare variables. I would advise you to use the former, as var
can cause problems with scoping, possibly introducing vulnerabilities to your software.
But what is a scope? Essentially, the “scope” of a variable is the block of code (indicated by pairs of curly braces - {}
) in which a variable exists. This could be a single function, file, or an entire codebase. Variables accessible everywhere in your code are usually called globals.
let
and const
are confined to the closest block in which they are defined, whereas var
is accessible to an entire function, and in some cases global by default - not great. Global variables are usually avoided, as anyone with access to our code can change them, which may effect other bits of our program.
The concept scope is complicated. Luckily, you don’t need to understand much about it when you start out! If you do want to learn more, there are lots of clever people who have written explanations of the concept:
- https://www.w3schools.com/js/js_scope.asp (Nice n’ simple)
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/let
- http://javascriptkit.com/javatutors/javascript-es6-let-const.shtml
Conditionals
Making decisions is an important function of any computer. We should be able to branch into alternative code pathways, and execute different code in different conditions (described by boolean expressions).
The simplest decision is whether or not to run a block of code. For this, we use an if
statement, which executes a block of code if a certain condition is true:
if (age == 18) {
console.log("Congrats! You're now an adult.");
}
Here’s a handy flowchart so you can see what’s going on:
Sometimes we want to do something when the condition is not true - an “else” condition:
And in code:
if (age == 18) {
console.log("Congrats! You're now an adult.");
} else {
console.log("You aren't 18.")
}
And, sometimes you want to check another condition if the first was false
.
This is where if else
comes in:
if (age == 18) {
console.log("Congrats! You're now an adult.");
} else if (age < 0) {
console.log("You aren't born yet!");
} else {
console.log("You aren't 18 and you're alive. Well done.")
}
Nice! We can now write conditional code. You’ll be a pro in no time!
If you fancy a different explanation and some more examples, check out https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/if...else
Loops
Sometimes we want to repeat ourselves. Let’s say we wanted to output “Hello, " and your name 10 times. Is this how we’d do it?
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
console.log("Hello, Tim");
Probably not. It’s quite messy, takes up a bunch of space, and is not very maintainable (imagine changing the name!)
A good thing to remember as you learn is to write DRY code (Don’t Repeat Yourself) 3. Repeated code is a good sign that there’s a better way. Avoid WET, repeated code (Write Everything Twice). Let’s refactor our code to make it less repetitive, by adding a variable to store the name, and a while
loop:
const name = "Tim";
let i = 0;
while (i < 10) {
console.log("Hello, " + name); // The + operator concatenates strings
i++;
}
If you run this, you’ll see that we get the same result, but our code looks a great deal better. We have a constant, name
, and a while
loop.
The loop is likely new to you, so let’s unpack it. Essentially, the loop will repeat while the condition is true
:
There are three important parts to our loop:
let i = 0;
- Creates an iterator variable calledi
(you can call it whatever you wish) and sets it to0
i < 10
- The boolean expression (condition) evaluated each loop iterationi++
- Really important! Without this,i
will remain the same, and our loop will go on forever (an infinite loop)i++
is shorthand for writingi = i + 1
ori += 1
- It adds one to
i
, then stores the result back into the variable
Another type of loop is the for
loop, which is more concise:
for (let i = 0; i < 5; ++i) {
console.log("I'm number", i);
}
Note that comma-separated arguments to console.log()
are separated by a space in the output. I’ve also used ++i
here, but note in this case, i++
behaves the same.
The code above should output:
I'm number 0
I'm number 1
I'm number 2
I'm number 3
I'm number 4
The syntax of a for
loop is based around what’s in those brackets:
for ([initialisation]; [condition]; [expression])
let i = 0;
- The initialisation step, where we set up our iterator like beforei < 5;
- The condition evaluated each loop iteration++i
- The expression updating our iterator variable
As you get more experienced, you’ll see what type of loop is best for each situation, but in most cases they are interchangeable, so practice with both!
You can learn more about loops here:
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/while
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for
Example: Guessing Game
You’ve learned enough that you can create your own text-based game! How cool is that‽
First, we need to create a file. While the console is great for learning and testing stuff out, it’s a bit annoying for anything permanent. Instead, you can create a JavaScript file with any plain text editor. Note that the “plain” bit is important - don’t create code in a word processor, as these programs add a bunch of other stuff into files that we don’t want!
You could use something like Notepad on Windows, TextEdit on macOS or GNOME Text Editor on Linux, but these programs aren’t really designed for writing code. Instead, you’ll want to use something like Sublime Text, Atom, or Visual Studio Code (what I’m using to write this!) All of these programs are free, so check them out and see what you think! There are also more advanced Integrated Development Environments (IDEs) available, but we won’t concern ourselves with those.
To start with, a HTML file will be used to embed our JavaScript code, attaching it to a web page that can be viewed in our browser. Hyper-Text Markup Language (HTML) is the language used to structure web pages, which is interpreted by web browsers. This is not a HTML tutorial, so we’re going to use simple code (not valid HTML). The following line attaches a “script.js” file located in the same directory as our HTML file:
<script src="script.js"></script>
Save this file into a directory (folder) on your computer, with the extension “.html”. I saved it as “index.html”. Open this file in your web browser, and you will see a blank page.
You can check it’s the right file by adding some text to the bottom:
<script src="script.js"></script>
Hello!
If you want, learn more about HTML here: https://www.w3schools.com/html/default.asp
Now, we add our JavaScript. Create a file called “script.js” and save it in the same directory as your HTML file. You can add a console.log()
in the file to check everything is properly linked. Check that they are both in the same directory if you have issues.
If you skip ahead, you can find my full solution, but you will gain little knowledge by just copying it. Programming is about problem solving, a skill that only comes with practice. You need a couple of building blocks, most of which you already have.
Building Block #1
const number = Math.floor(Math.random() * 10) + 1;
Before you start, you will want to generate a random number. For this, we can use the Math.random()
method. Note that the result is not actually random 4, but it’s good enough for us.
We want a random number between 1 and 10. Math.random()
generates a random number between 0 and 1, which we multiply by 10, so we get a number between 0 and 10 (not including 10). Try calling Math.random()
and Math.random()*10
a few times in your console to see the result. We then round down (Math.floor()
), to get an integer (whole number). We then add 1 so we get numbers 1-10, not 0-9.
How did I work this out? I searched the internet. There is nothing wrong with using Google, DuckDuckGo, Bing, or whatever your wish to lookup stuff. Developers don’t need to have the best memories, and use search engines all the time! You can do this if you ever get stuck :)
Building Block #2
const guessInput = prompt("Take a guess!");
const guess = Number(guessInput);
The second thing you need to know is how to get player input. Typically you don’t use prompt()
much, but it’ll do for us. We get user input as a string, then convert it to a number, stored in the guess
variable. Note you could do this in one line, by nesting the prompt()
inside the call to Number()
.
You now have all you need to write the program - conditionals, loops, random numbers and user input. Give it a shot then we’ll discuss a possible solution. Remember that one problem can have many solutions, some better than others.
If you need some help getting started, look at my algorithm below. It is represented as pseudo-code (code not in any particular language.) Once you have this, it’s easier to translate the idea into a concrete program.
while <player has guesses left>
guess = <player input>
if guess is correct
tell player
if guess is too high
tell player
if guess is too low
tell player
Solution
Okay, let’s look at my solution:
const MAX_GUESSES = 3;
const number = Math.floor(Math.random()*10)+1;
let guesses = 0;
while (guesses < MAX_GUESSES) {
const guess = prompt("Guess my number between 1 and 10");
if (Number(guess) === number) {
alert("You guessed correctly!");
break;
} else if (guess > number) {
alert("Too high!");
} else {
alert("Too low!");
}
guesses++;
}
It’s not the best solution, and you will likely have come up with something a little different. There is a limit to the number of guesses (MAX_GUESSES
), but you could also allow infinite guesses, exiting when the user guesses correctly. I used alert()
to keep consistent with the prompt
s, but you could also use console.log()
or document.write()
(to write text directly to the page).
Note the constant guess
only exists in the scope of each loop iteration, so it is immutable only in that scope, and recreated every loop round.
Functions
You’ve written your first program, and hopefully you understand you need only a few building blocks to assemble some great code. That’s what functions are - building blocks of code that you can reuse. Some take different parameters each call to yield different results.
Head back to your browser console or stay with the file, and follow along!
When we were using console.log()
earlier, I pointed out that you see the return value undefined
in the console before the text is output. Every function/method has some return value, the default being undefined
, which means no result was returned.
To clear up the terminology, a function looks like this:
function myFunction() {
// Some code that does something
console.log("I'm calling this method inside my function!");
}
…and a method is a function inside an object, which we’ll come to later.
If you run the above code, nothing will happen. That’s because this is a function definition, and the code is not executed. We create a new function, named myFunction
, and inside the curly braces define its behaviour for when it’s called.
We can call our function with its name, running the code defined above:
myFunction();
This function call can be treated like any statement. It can be nested inside a conditional, a loop, or another function.
Functions can also take parameters:
function add(num1, num2) {
let sum = num1 + num2;
return sum;
}
const result = add(3, 4);
console.log(result);
No prizes for guessing what this does. We take two numbers and return their sum.
num1
and num2
are formal parameters, which are replaced by the actual parameters (3
and 4
) in the function call. This is really useful, as we can call the same block of code many times with different parameters.
Most of the time, functions can be copied between programs so you can reuse them in future projects. Third-party libraries are also available which provide useful functions written by others that you can use in your own projects.
Go ahead and build some of your own functions to see what you can come up with. Here’s a function that would be handy for our game:
function randomNumber(min, max) {
return Math.floor(Math.random()*max)+min;
}
We could reuse this function in future programs to generate random integers.
Objects
If you come from an object-oriented language such as Java, it’s important to note that “objects” in JavaScript are a little different. Objects in JavaScript store key-value pairs:
const myObject = {
aKey: "This is a string value",
aNumber: 37,
Person: {name: "Jeff", age: 37} // An object inside an object?! Yes we can!
};
const val = myObject.aNumber;
console.log(val);
You can access these values with the dot notation (object.key
), shown above. Note that keys can’t have spaces.
You can also use strings as keys, letting you use any characters that can live inside a string! You access these values using subscript notation (object["key"]
).
const stringObj = {
"a string": "blah blah blah...",
"num": 25,
"key_234": false
};
const val = stringObj["num"];
console.log(val);
You can store a lot of things in objects, even functions! Such functions are called methods, and are written a bit differently:
const person = {
name: "Steve",
age: 45,
log: function () {
console.log("My name is", this.name, "and I am", this.age, "years old");
}
};
person.log(); // Output: My name is Steve and I am 45 years old
We use the this
keyword to access the stuff inside the object when we’re writing methods. this
is a contextual variable, so it represents different things in different places in our program. In this case, this
represents our object. Because the this
keyword changes, it can be a bit tricky to understand, and we won’t discuss it at length, but you can read more about it here: https://www.w3schools.com/js/js_this.asp.
You can find out more about objects here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object
Arrays
Arrays are an ordered collection of items. In JavaScript, arrays are actually objects, where keys are numbers. You can access its contents using the subscript syntax, but instead of a string you use the numeric index, starting from 0
:
const arr = [2, 4, -3, 32, 11, -354];
// Retrieve item
console.log(arr[2]); // -3
// Replace item
arr[0] = 37;
Remember that an index of 0
indicates we’re looking at the first item!
Here are some useful methods you can call on arrays:
// Add an item to the end
arr.push(25);
// Get the length
arr.length(); // 7
You can use a loop to iterate through all the items in an array like so:
for (let i = 0; i < arr.length(); i++) {
console.log(arr[i]);
}
You can find out more about arrays here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array
Debugging
Some final key tips for debugging programs, if you have issues:
- Use
console.log()
to output the state of variables or the result of expressions - is that what you expected? - Use rubber duck debugging! Explain your problem to an inanimate object (like a rubber duck, fluffy toy, your friend…) This is often surprisingly helpful!
- Check you have finished statements with semicolons as appropriate.
- Check if you have closed brackets (
()
) and blocks of code ({}
). Every opening bracket should have a matching closing bracket. - Make sure you are using
=
for variable assignments and===
for comparisons.
Conclusion
Hopefully now you feel comfortable with the building blocks, and can start creating some simple programs. You might want to check out the W3Schools JavaScript tutorial for some cool stuff you can do with JavaScript, such as making buttons, matching regular expressions and manipulating the HTML Document Object Model (DOM) or Browser Object Model (BOM). I look forward to seeing what you make!
Any corrections or comments are welcomed!