Raspberry Pi Traffic Lights
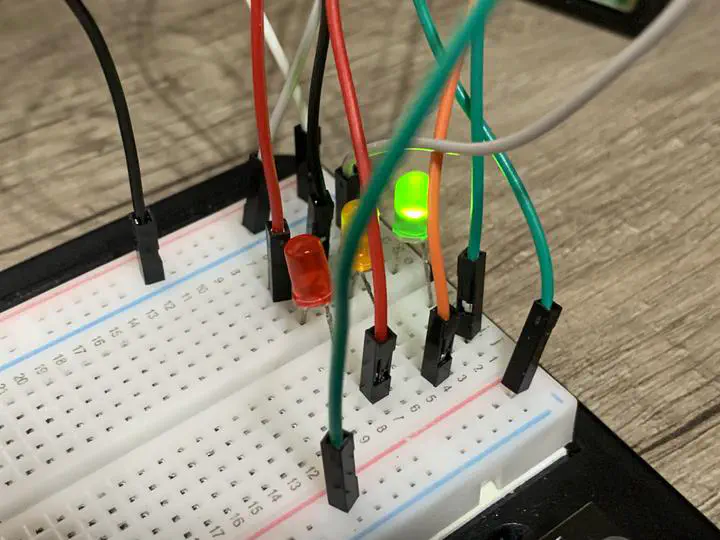
The classic hardware project to test out the GPIO 1 pins on my old Raspberry Pi! Made slightly more “advanced” by simulating two types of crossing depending on whether or not the IS_PUFFIN
flag is set.
from gpiozero import LED
from time import sleep
IS_PUFFIN = True
GREEN_LENGTH = 10
green = LED(17)
amber = LED(22)
red = LED(27)
def reset():
green.off()
amber.off()
red.off()
def stop():
reset()
amber.on()
sleep(1)
amber.off()
red.on()
sleep(5)
def go_puffin():
reset()
red.on()
amber.on()
sleep(1)
red.off()
amber.off()
green.on()
sleep(GREEN_LENGTH)
def go_pelican():
reset()
red.on()
sleep(1)
red.off()
for i in range(5):
amber.on()
sleep(0.5)
amber.off()
sleep(0.5)
green.on()
sleep(GREEN_LENGTH)
print("Running...")
while True:
go_puffin() if IS_PUFFIN else go_pelican()
stop()
Testing
Future Work
- Simulation of “traffic” and “pedestrians” using LEDs or similar to display randomised traffic patterns
- Program a car using image recognition to detect the red light and stop accordingly